Keeping your application safe is just as important as building cool features. Laravel security helps protect your site from hackers, data leaks, and other online threats. In this guide, we’ll explore simple, real-world tips to secure your Laravel app—so even beginners can understand and apply them with confidence.
1. 🔒 Always Use HTTPS (Lock Your House)
What it means: Use https://
instead of http://
.
Why: Just like how a lock keeps your house safe, HTTPS keeps the data safe when someone visits your site. It stops people from spying or stealing info like passwords.
What is HTTPS?
It’s like sending a sealed envelope instead of a postcard. HTTPS makes sure nobody can read your messages while they travel over the internet.
Without HTTPS
If your site is on HTTP and someone connects using public Wi-Fi (like at a coffee shop), a hacker can see the data — like passwords and messages!
✅ Example:
- Get an SSL certificate
- In your
.env
file:
APP_URL=https://yourdomain.com
Also, make sure your web server (like Nginx) redirects http
to https
.
2. 🔑 Hide Your Secret Key (Don’t Show the Key Under Your Mat)
What it means: Don’t share .env
file or API keys anywhere.
Why: Your .env
file has secret stuff (like DB username and password, Email service credentials, aravel’s secret APP_KEY
). If someone gets it, it’s like giving them the key to your house.
✅ Example: Never push .env
to GitHub, add this to your .gitignore
so it’s never uploaded:
.env
And never do this:
return response()->json(env('DB_PASSWORD')); // ❌ Don't expose secrets!
3. 👮♂️ Use Laravel’s Built-in Auth (Use Laravel’s Security Guards)
What it means: Use Laravel’s Auth
system instead of making your own.
Why: Laravel already built strong locks and alarms. Trust them — don’t try building your own unless you’re a locksmith (security expert).
Laravel Auth handles all the safe login stuff:
- Hashed passwords
- Session handling
- Login throttling (stop brute-force attacks)
✅ Example:
php artisan make:auth # For Laravel UI
Or use Laravel Breeze, Jetstream, or Fortify for modern solutions.
In Laravel Breeze for clean and modern auth:
composer require laravel/breeze --dev
php artisan breeze:install
npm install && npm run dev
php artisan migrate
That’s it! Login, register, and reset password just work — safely.
4. 🧼 Validate User Input (Don’t Let Strangers Bring Garbage Inside)
What it means: Always check what users are sending in forms.
Why: If you don’t check, they could send harmful code (like a virus) that breaks your app. Laravel’s Request
validation helps you clean things up.
Letting users enter anything they want is risky! They might:
- Submit broken data
- Send malicious code (XSS)
- Trick your app
✅ Example:
$request->validate([
'email' => 'required|email',
'password' => 'required|min:8',
]);
Never trust what users type. Always clean it!
5. 🧯Protect Against SQL Injection (No Sneaky Messages to Your Database)
What it means: Use Eloquent or Laravel’s query builder instead of writing raw SQL.
Why: If you let people type into a form and that input goes straight into your database, they could send a message to delete everything. Laravel protects you if you use it right.
What is SQL Injection?
It’s when a hacker puts evil SQL code into your query, like:
'OR 1=1 --
Without protection:
//This is dangerous!
$email = $_GET['email'];
$users = DB::select("SELECT * FROM users WHERE email = '$email'");
A hacker could use:
?email=' OR 1=1 --
…and get every user’s data.
Use Eloquent (safe):
$user = User::where('email', $email)->first();
6. 🛑 CSRF Protection (Don’t Let Fake Visitors Trick You)
What it means: Laravel automatically adds a CSRF token to forms.
Why: Imagine someone knocks on your door pretending to be your friend. CSRF tokens help you know who’s really who — like a secret handshake.
✅ Example:
Every Laravel form already has a hidden CSRF token:
<form method="POST" action="/login">
@csrf
<!-- input fields -->
</form>
It’s like a secret handshake between your form and the server.
Without CSRF: A hacker could send a fake form to update your profile without you knowing!
7. 🧱 Set Proper Permissions (Only Open Rooms They Should Enter)
What it means: Make sure users only see what they’re allowed to.
Why: Just like your guests shouldn’t go into your bedroom, users shouldn’t see admin stuff unless they’re admins. Use policies
or gates
.
✅ Example:
Gate::define('edit-post', function ($user, $post) {
return $user->id === $post->user_id;
});
And use it like:
@can('edit-post', $post)
<a href="/edit">Edit</a>
@endcan
8. 🚫 Hide Error Messages (Don’t Tell Robbers Where the Safe Is)
What it means: Never show detailed error messages in production.
Why: Error messages can leak info like your file paths, database names, etc. That’s like telling burglars where your safe is hidden.
Set this in .env
:
APP_DEBUG=false
If APP_DEBUG=true
:
Hackers can figure out how your app works and where to attack.
9. 📦 Keep Laravel Updated (Upgrade Your Locks)
What it means: Regularly update Laravel and packages.
Why: Hackers find new tricks all the time. Laravel releases updates to stop them. If you don’t update, you leave the backdoor open.
✅ Example:
Check if you’re using the latest version:
composer outdated
Then update:
composer update
10. 🔐 Set a Strong APP_KEY
(Your Master Key)
What it means: In your .env
file, there’s a key called APP_KEY
. Laravel uses this to lock and unlock data.
APP_KEY=base64:yourVeryLongSecretKeyHere
Why: Imagine this is your master key that locks all the important boxes in your app — like passwords, sessions, and encrypted data.
If this key is missing or weak, it’s like leaving your house unlocked.
What to do:
- After creating a Laravel project, always run:
php artisan key:generate
- This command sets a strong, random
APP_KEY
in your.env
file. - Never share this key with anyone or commit it to GitHub.
Security is like brushing your teeth — do it daily, not just when something breaks!
Summary:
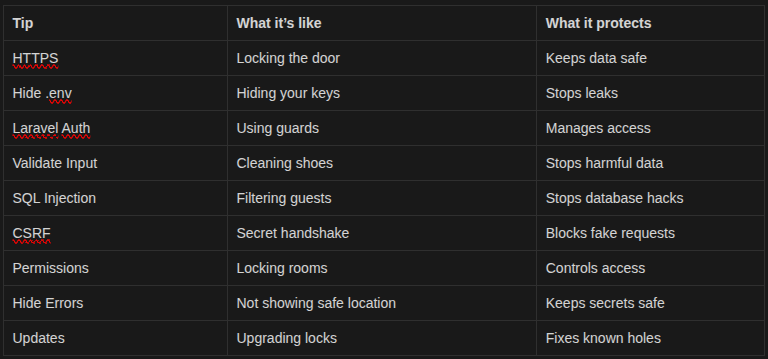
For more insightful tutorials, visit our Tech Blogs and explore the latest in Laravel, AI, and Vue.js development!